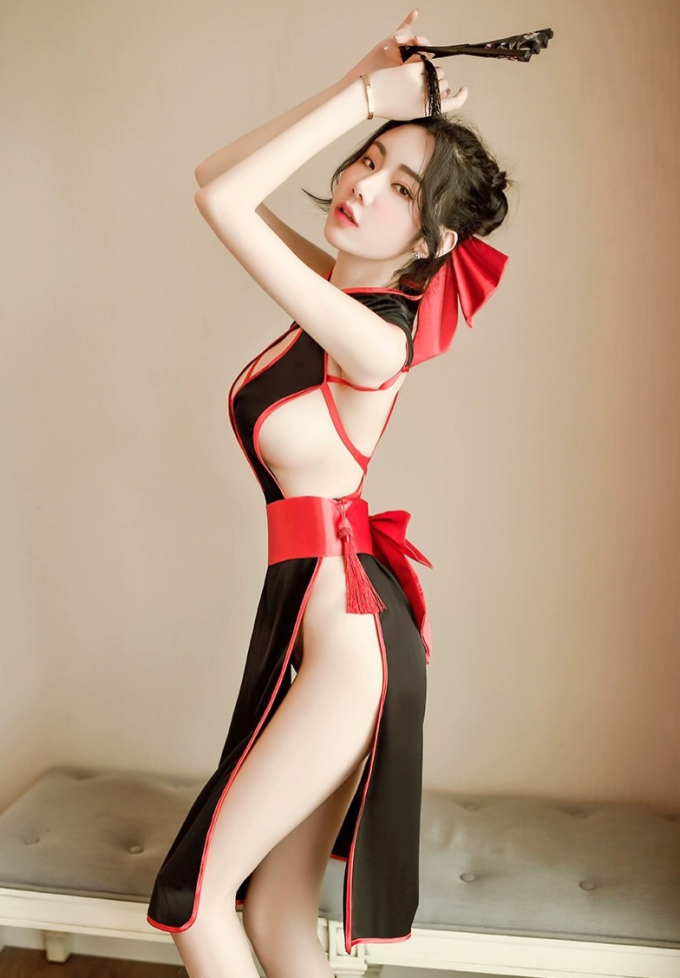
用Python做一个俄罗斯方块小游戏:手把手教学版(附详细图解)
-
2025-02-20
-
点评
0
- 类型: 应用软件
- 大小: 49
- 语言: 中文
- 系统: v10.32.39
- 发布时间:
- 推荐指数: ★★★★★
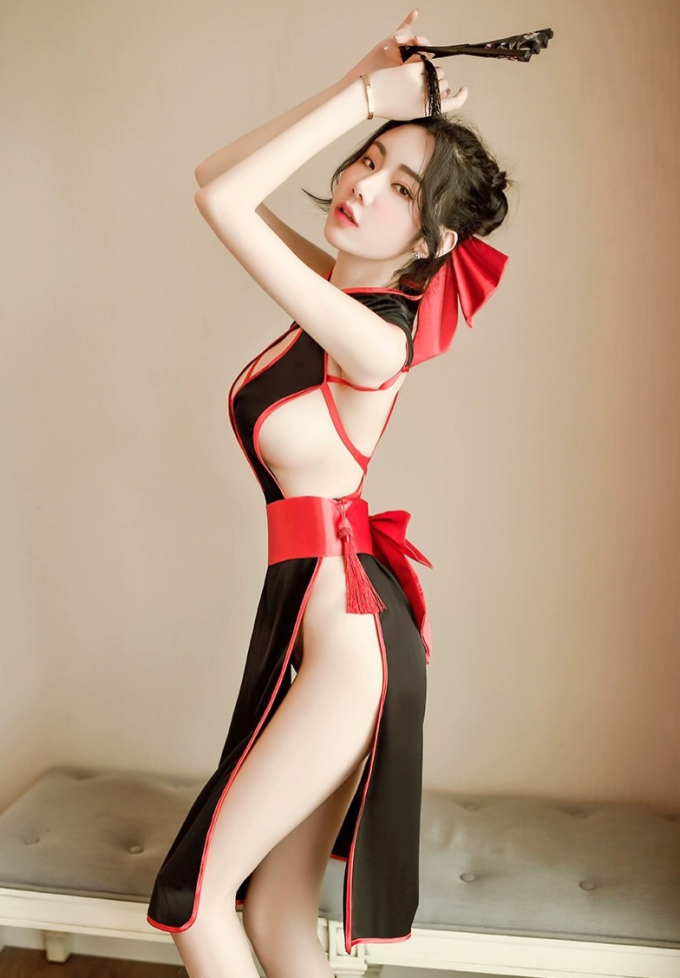
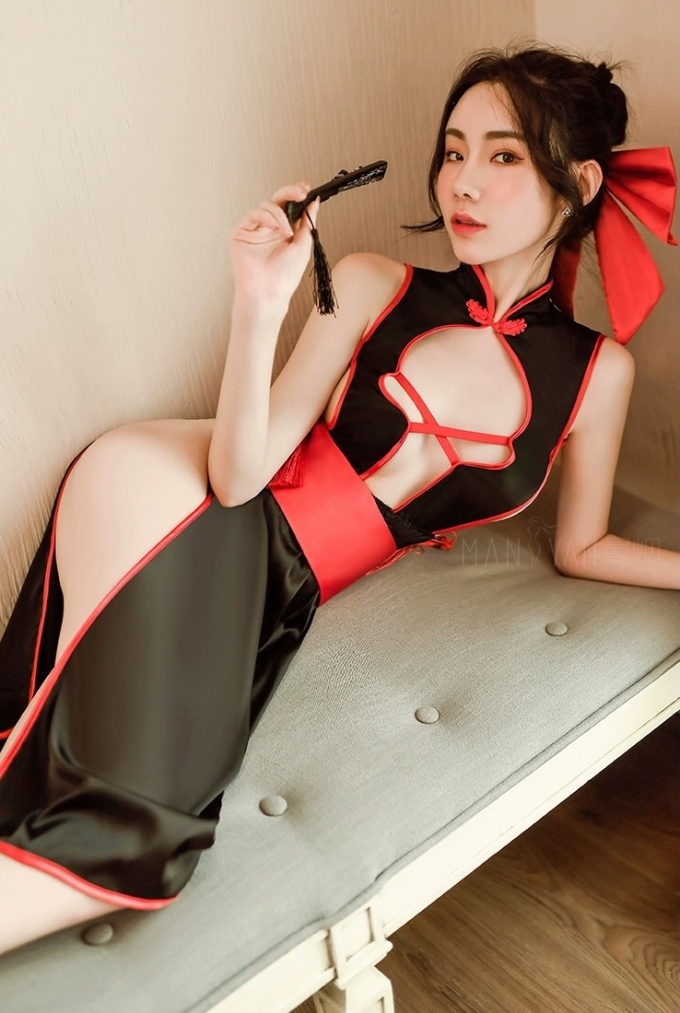
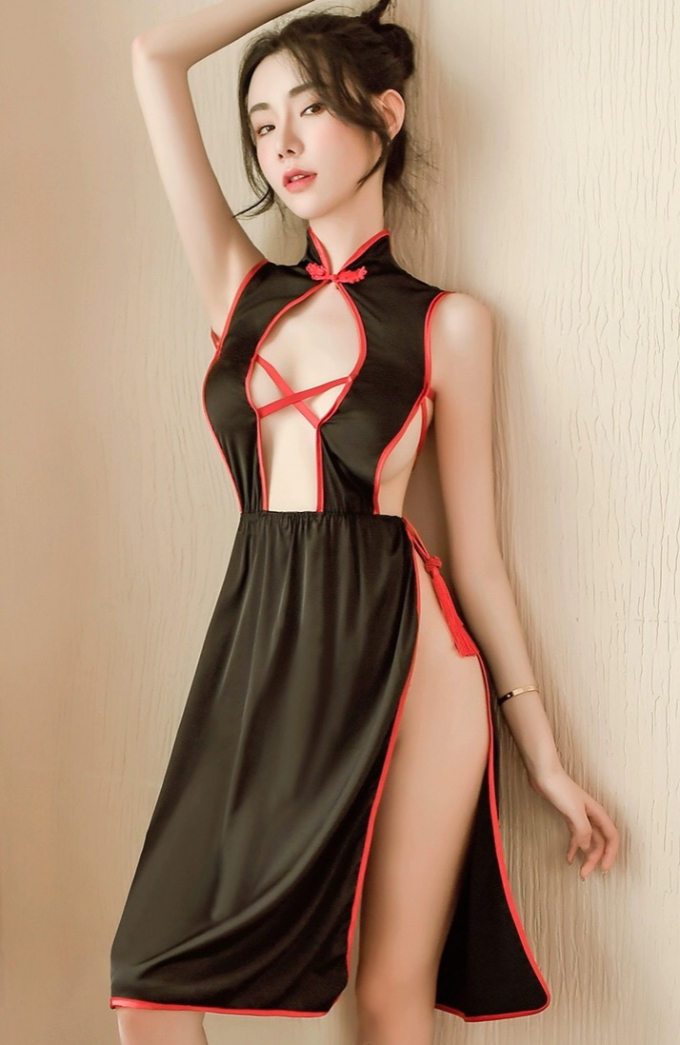
最终实现界面
一、开发环境搭建(10分钟)1.1 安装Python1.2 安装PyGame库
pip install pygame
二、创建游戏窗口(20分钟)2.1 初始化基础代码
新建 tetris.py 文件,输入以下代码:
import pygame
# 初始化游戏引擎
pygame.init()
# 窗口设置
WIDTH = 300 # 窗口宽度
HEIGHT = 600 # 窗口高度
GRID_SIZE = 30 # 每个小方块边长
# 创建窗口
screen = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption("我的俄罗斯方块")
# 游戏主循环
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
screen.fill((0, 0, 0)) # 用黑色填充背景
pygame.display.update() # 更新画面
pygame.quit()
2.2 运行测试
图2
2.3 常见问题解决三、创建方块系统(30分钟)3.1 定义方块形状
在代码开头添加以下内容:
# 7种经典形状(用0和1表示)
SHAPES = [
[[1, 1, 1, 1]], # I型
[[1, 0, 0], [1, 1, 1]], # L型
[[0, 0, 1], [1, 1, 1]], # J型
[[1, 1], [1, 1]], # O型
[[0, 1, 1], [1, 1, 0]], # S型
[[0, 1, 0], [1, 1, 1]], # T型
[[1, 1, 0], [0, 1, 1]] # Z型
]
# 经典颜色配置(与形状顺序对应)
COLORS = [
(0, 255, 255), # 青色
(255, 165, 0), # 橙色
(0, 0, 255), # 蓝色
(255, 255, 0), # 黄色
(0, 255, 0), # 绿色
(255, 0, 255), # 紫色
(255, 0, 0) # 红色
]
3.2 创建方块类
在代码中添加类定义:
class Game:
def __init__(self):
self.board = [[0]*(WIDTH//GRID_SIZE) for _ in range(HEIGHT//GRID_SIZE)]
self.new_piece() # 创建新方块
def new_piece(self):
"""生成新方块"""
self.current_shape = random.choice(SHAPES)
self.current_color = random.choice(COLORS)
self.x = (len(self.board[0]) - len(self.current_shape[0])) // 2 # 居中显示
self.y = 0 # 从顶部开始
3.3 绘制方块
修改主循环中的绘制部分:
# 在while循环内添加:
# 绘制当前方块
for y, row in enumerate(game.current_shape):
for x, cell in enumerate(row):
if cell: # 只绘制值为1的格子
pygame.draw.rect(screen, game.current_color,
((game.x + x)*GRID_SIZE,
(game.y + y)*GRID_SIZE,
GRID_SIZE-1, GRID_SIZE-1)) # -1是为了显示网格线
四、实现方块移动(25分钟)4.1 处理键盘事件
在主循环的事件处理部分添加:
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
game.x -= 1 # 左移
elif event.key == pygame.K_RIGHT:
game.x += 1 # 右移
elif event.key == pygame.K_DOWN:
game.y += 1 # 加速下落
4.2 添加碰撞检测
在Game类中添加方法:
def check_collision(self, dx=0, dy=0):
"""检测是否碰撞 dx:水平偏移 dy:垂直偏移"""
for y, row in enumerate(self.current_shape):
for x, cell in enumerate(row):
if cell:
new_x = self.x + x + dx
new_y = self.y + y + dy
# 边界检测
if new_x < 0 or new_x >= len(self.board[0]):
return True
if new_y >= len(self.board):
return True
# 已有方块检测
if self.board[new_y][new_x]:
return True
return False
4.3 限制移动范围
修改键盘事件代码:
if event.key == pygame.K_LEFT and not game.check_collision(-1, 0):
game.x -= 1
elif event.key == pygame.K_RIGHT and not game.check_collision(1, 0):
game.x += 1
elif event.key == pygame.K_DOWN and not game.check_collision(0, 1):
game.y += 1
五、实现自动下落与堆积(25分钟)5.1 添加自动下落
在主循环前初始化计时器:
game = Game()
fall_time = 0
fall_speed = 500 # 下落间隔500ms
clock = pygame.time.Clock()
在循环内添加:
delta_time = clock.get_time()
fall_time += delta_time
if fall_time >= fall_speed:
if not game.check_collision(0, 1):
game.y += 1
fall_time = 0
else:
# 固定方块并生成新方块
game.merge_piece()
game.new_piece()
5.2 实现方块堆积
在Game类中添加:
def merge_piece(self):
"""将当前方块固定到棋盘"""
for y, row in enumerate(self.current_shape):
for x, cell in enumerate(row):
if cell:
self.board[self.y + y][self.x + x] = self.current_color
六、添加消除与游戏结束判断(20分钟)6.1 行消除功能
在Game类中添加:
def clear_lines(self):
"""消除满行"""
lines_to_clear = []
for i, row in enumerate(self.board):
if all(row): # 所有格子都有颜色
lines_to_clear.append(i)
# 删除满行并添加新行
for i in lines_to_clear:
del self.board[i]
self.board.insert(0, [0]*len(self.board[0]))
6.2 游戏结束判断
在合并方块后添加:
if game.check_collision():
print("游戏结束!")
running = False
七、最终调试与运行(15分钟)7.1 完整代码整合
确保所有代码片段按正确顺序组合,最终代码应与第一节的完整代码一致
7.2 测试各个功能7.3 常见问题处理